Components of python programs full details
Now that you know how to write simple Python programs and how to execute them on different implementations, it’s time to take a closer look at the that make up a Python program. Since you have probably already done some form of programming, it’s safe to describe programming as manipulating variables, since this is what all programs do regardless of their host language.
It’s worth remembering throughout this chapter, and indeed during any Python programming exercise, that Python deals with objects. Although objects in Python may appear to be similar to the variables used in other languages (and we’ll be making those comparisons in this chapter), all Python variables are, in truth, objects.
The Python language follows the same basic principles as many others:
•An individual application is made up of a number of files, which Python calls modules.
•Each module is composed of a number of statements.
•The statements create, use, and modify variables, and Python creates object variables.
This chapter describes the two main areas that make up a Python program, Built-in Object Types and the Statements. Since the logical flow is from the lowest common denominator, the object, to the highest, a module, we’ll look at objects first and then look at statements Chapter t looks at modules in-depth, after you’ve mastered the basic components of Python language.
Built-in Object Types
All languages support a number of built-in variable types. These are the building blocks used for all the other variable types available within the language. By combining or extending the basic variables, you can create some quite complex variables. For example, in C there is a basic type called char, which is a single character. By defining a char array, you create a text string, and by defining a two-dimensional array, you create an array or a string of strings.
Python supports a number of built-in object types Unlike C, which approaches the problem of variables by supporting the lowest common denominator, Python supports a number of high-level variables that are more practical for most program solutions. Unlike other languages, Python variables are actually objects. By using objects at the basic storage level, the overall language is object-based. This has a number of advantages. You can use the same methods and functions to operate on different types of objects. Furthermore, because new objects inherit object methods, you can continue to use the same methods in the more complex objects that you create. You’ll see some examples of this later in this chapter.
Just like other scripting languages, Python handles the creation, and memory allocation. and the access routines that enable you to use the objects. This is the same model as offered by Visual Basic (VB) and Perl, but it’s different from C. where you are responsible for allocating memory for the variables and information you need to store.
Python actually supports a wide variety of built-in object types that help to make your programs easier to write. Many of the basic types offered by Python would actually require a separate library or development process to be implemented before the object could be used. By providing these built-in, optimized versions, you can start programming right away with Python without worrying about the complexities of the objects you are using.
The use of built-in object types also has another advantage. The code for using built-in object types is highly optimized (and debugged) and has been developed over a number of years to be as efficient as possible. Although it is possible to write your own routines for implementing the same objects within your own applications, it is difficult to improve upon the versions supplied as built-in objects with the Python interpreter.
Python Objects and Other Languages
Python supports five main built-in object types and an external data type that is accessible just like the built-in objects. The built-in objects are Numbers, Strings, Lists, Dictionaries, and Tuples.
The primary external data type supported by Python is the file. Although it may seem odd to include files as a data type, Python supports access to files in much the same way that it supports access to the built-in object types. You’ll see how this works and how the file and object types compare later in this chapter.
Some of the built-in object types will be familiar to you, such as numbers and strings, and possibly lists, although Python supports a few different options on some of these objects. The other object types are probably unfamiliar to you with their current names. Table 3-1 offers a comparison of Python’s built-in object types with data types offered by other languages.
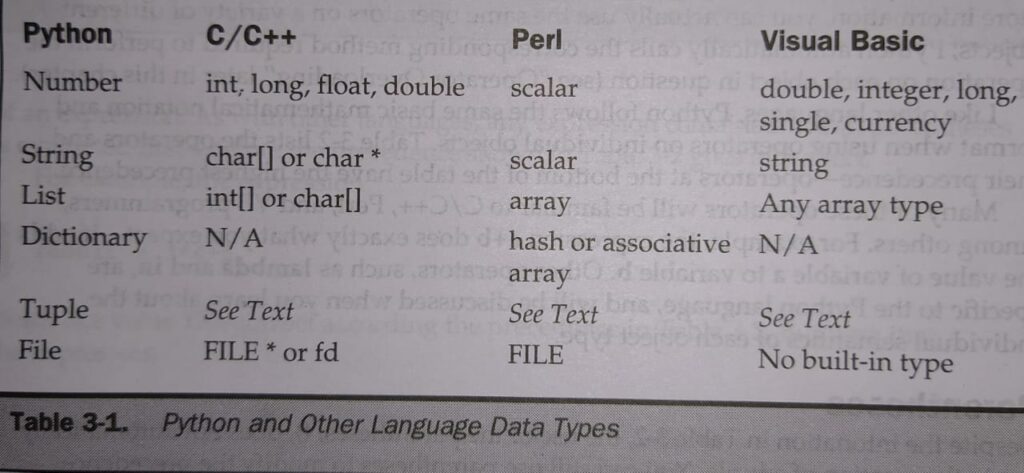
The tuple is a special type of list that cannot be modified. Since it’s similar to a list, there is no reason why you can’t use a normal array in C/C++, Perl, or VB. See the section “Tuples” later in this chapter for details on how tuples differ from normal lists. Unlike other languages, it is the way in which the information is assigned to the object that defines the object type used to store the information In C, you must explicitly define the type of the variable you are creating. In some languages, including Perl, the leading character in the variable name tells the language’s interpreter what the object type is With Python, the interpreter examines the information being placed into the object and then creates or modifies the object to match the new type.
To see this in action, the following code shows different ways in which you can create objects operation is called an assignment since you assign a value to a variable:-
integer = 12345
float = 123.45
string - 'Hello'
list [1, 'Two', 3]
dictionary= {'one':1, 'two' :2, 'three':3}
Tuple = (1, 'two', 3)
In each case, Python identifies the information you are assigning to the variable and uses the correct object type for the data that needs to be stored. You’ll learn more about the semantics of the assignment process when we describe each individual object type.
Operator Basics
It’s impossible to talk about objects without also talking about the operators that can be used to operate on the objects. Because Python uses objects rather than variables to store information, you can actually use the same operators on a variety of different objects, Python automatically calls the corresponding method required to perform the operation on each object in question (see “Operator Overloading” later in this chapter). Like other languages, Python follows the same basic mathematical notation and format when using operators on individual objects. Table 3-2 lists the operators and their precedence-operators at the bottom of the table have the highest precedence Many of these operators will be familiar to C/C++, Perl, and VB programmers, among others. For example, the expression a+b does exactly what you expect it adds the value of variable a to variable b. Other operators, such as lambda and in, are specific to the Python language and will be discussed when you learn about the individual semantics of each object type.
Parentheses
Despite the intonation in Table 3-2, the use of the parentheses, (), does not automatically imply the creation of a tuple. You can still use parentheses to modify the precedence
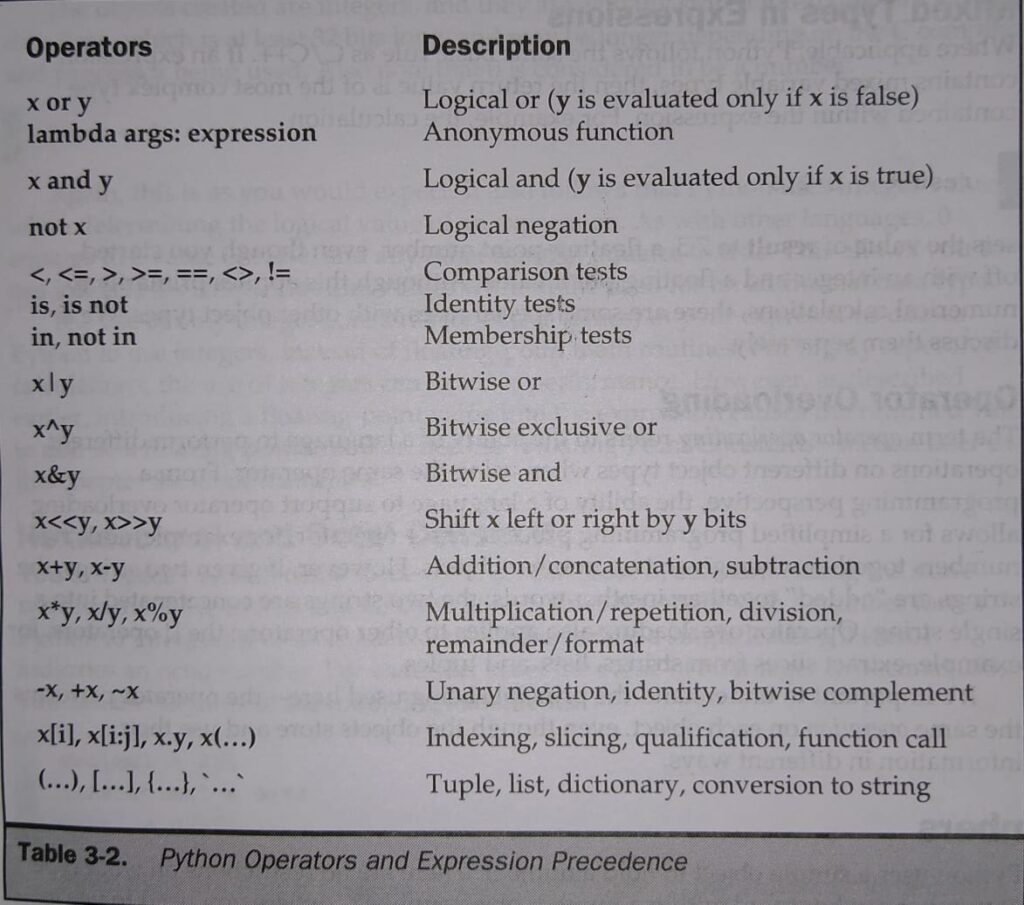
of an expression. As with other languages, any expression contained within parentheses is evaluated first before the precedence shown in table 3-2 goes into effect.
For example, the expression
result = 3+4+5
yields the value 17-correct according to the precedence in table 3-2. But note that the expression.
result = 3*(4+5)
return a Value of 27.
Mixed Types in Expressions
Where applicable, Python follows the same basic rule as C/C++. If an expression contains mixed variable types, then the return value is of the most complex type contained within the expression. For example, the calculation
result = 3*2.5
sets the value of result to 75, a floating-point number, even though you started off with an integer and a floating point value. Although this applies primarily to numerical calculations, there are some special cases with other object types. We’ll discuss them separately.
Operator Overloading
The term operator overloading refers to the ability of a language to perform different operations on different object types when using the same operator. From a programming perspective, the ability of a language to support operator overloading allows for a simplified programming process. The operator, for example, adds two numbers together when given two number objects. However, if given two strings, the strings are “added together in other words; the two strings are concatenated into a single string Operator overloading also applies to other operators; the II operators, for example, extract slices from strings, lists, and tuples.
It’s important to understand the principle being used here the operator performs the same operation on each object, even though the objects store and use their information in different ways.
Numbers
Python uses a simple object to hold a number. There are no restrictions on what type of the number can be stored within a number object, unlike C, where you need to use a different data type to store the integer and floating-point numbers. In addition to these basic types, Python also supports complex numbers and arbitrarily large integers As you already know. Python creates an object using the object type as determined from information that is assigned to the object when it is created. With number objects. the format of the number determines the method in which the information is stored. Therefore, you need to know how to introduce numerical constants into your Python programs.
Integer Constants
You can create integer number objects by supplying a sequence of numbers, as in the following example:
number = 1234
number = -1234
The objects created are integers, and they are actually stored internally as a C-long data type, which is at least 32 bits long and may be longer depending on the C compiler and processor being used. Also note that is considered to be a number:-
zero = 0
Again, this is as you would expect. It also follows that python uses integer values when determining the logical value of an expression. As with other languages. 0 equates to a value of false, and any other number equates to true. This allows you to use integers for simple Boolean values without the need for an additional data type. The use of only integer constants (or object values) in your expressions causes Python to use integers, instead of floating-point math routines. For highly repetitive calculations, the use of integers can increase performance. However, as described earlier, introducing a floating point value into the expression causes the returned value to also be a floating-point number. See the “Floating-Point Constants” section later in this chapter for some examples.
Hexadecimal and Octal Constants
You can specify hexadecimal (base 16) and octal (base 8) constants using the same notation available in Perl and C/C++. That is 0x or 0X prefixed to a number forces Python to interpret it as a hexadecimal number, while a single leading 0 (zero) indicates an octal number. For example, to set the value of an integer to decimal 255, you can use any one of the following statements:
decimal = 255
hexadecimal = 0xff
octal = 0377
Since these are simply integers, Python stores them in the same way as decimal integers. If you actually want to print an integer in octal or hexadecimal format, there is an interpolation method shown for formatting string objects that are described later in this chapter.
Long Integers
The built-in basic integer type is limited to a storage width of at least 32 bits. This means that the maximum number that you can represent is 2³¹-1 since you must use one bit to allow for negative numbers. Although for many situations this is more than enough, there are times when you need to work with long integers. Python supports arbitrarily long integers-you can literally create an integer one thousand digits long and use it within Python as you would any other number.
To create a long integer, you must append a 1 or L to the end of the number constant, as in the following example:-
long = 123456789123456789123456789123456789123456789L
Once you have created a long integer, you can execute expressions as if they were normal numbers-the Python interpreter handles the complexities of dealing with the super-sized number. For example, the statements
long =123456789123456789123456789123456789123456789123456789L
print long+1
produce the following output:
1234567891234567891234567891234567891234567891234567890L
Or you can continue to do long integer math:
long= 1234567891234567891234567891234567891234567891234567890L
print long+87654321987654321987654321987654321987654321987654321L
Which generates
21111111111111111111111111111111111111111111111111110L
Although Python uses these long integers as if they were normal integer values, the interpreter has to do a significant amount of extra work to support this option, even though support for such large numbers is written in C. If you can, use the built-in integer or floating paint types in preference to using long integer math.
Floating-Point Constants
Python supports the normal decimal point and scientific notation formats for representing floating point numbers For example, the following constants are all valid:-
number= 1234.5678
number= 12.34E10
number= -12.34E-56
Internally, Python stores floating-point values as C doubles, giving the objects as much precision as possible. Note that there is no “long” floating-point variable. Remember that floating point or mixed floating point and integer expressions return floating point values The easiest way to demonstrate this is to show the output from the same simple calculation-one using integer constants and the other floating-point constants:-
>>> print 5/12
0
>>> print 5.0/12
0.416666666667
You can see from the preceding example that the first expression return 0- the rounded-down version of 5/12. The second expression prints the expected decimal fraction.
For those situations where you are not using constants and therefore don’t have overall control of the object types you are using for your calculations, you can force an implied integer status to the expression supplied to the function. See the section “Type Conversion” at the end of this section.
Complex Number Constants
Python employs the normal notation for supporting complex numbers the real and imaginary parts are separated by a plus sign, and the imaginary number uses a single j or J suffix. For example, the following are examples of complex number constants:-
cplx =1+2j
cplx =1.2+3.4j
Python uses two floating-point numbers to store the complex number, irrespective of the precision of the original. Because complex math on expressions that include complex numbers.
Numeric Operators
Most of the operators in Table 3-2 apply to numbers. Table 3-3 contains a more explicit list of numeric operators used for calculations-these are all the familiar mathematical operations.
Operation | Description |
x+y | Add x to y |
x-y | Subtract y from x |
x*y | Multiply x by y |
x/y | Divide x by y |
x**y | Raise x to the power of y |
x%y | Modulo (returns the remainder of x/y) |
-x | Unary minus |
+x | Unary plus |
There are also a series of shift and bitwise operators that can be used for binary and bit math these are listed in Table 3-4. Note that these operators can only be applied to integers; trying the operations on a floating-point number raises an exception. In addition to these base operators, a series of augmented assignment operators was introduced with Python 20. For example, you can rewrite the fragment
a =a +5
using an augmented assignment operator, as
a +=5
More clearly, the expression
x= x + y
can be rewritten as
x+=y
The following is the full list of augmented assignment operators; the effects of these operators are the same as for the base operators listed in Table 3-4:-
+= -= /= %= **= <<= >>= &= ^= |=
Operation | Description |
x<<y | Left shift (moves the binary form of x, y digits to the left), for example, 1 << 1=4 |
x>>y | Right shift (moves the binary form of x, y digits to the right), for example, 16>>2=4 |
x & y | Bitwise and |
x | y | Bitwise or |
X^y | Bitwise exclusive or (xor) |
~x | Bitwise negation |
Numeric Functions
In addition to the operators mentioned in Table 3-2 earlier in this chapter, Python also has a small set of built-in functions that operate directly on numerical objects See Table 3-5 for a list of these functions. Note that this list does not cover those functions that convert objects between different types; the section Type Conversion later in this chapter explains those functions.
There are more numeric functions and some common constants defined in the math module, they are described in Chapter 10.
Function | Description |
abs(x) | Returns the absolute (numerical) value of a number. ignoring any signage. If the x is a complex number, the magnitude is returned. |
coerce(x,y) | Translate the two numbers x and y into a common type, using the normal expression rules, returning the two numbers as a tuple. For example, the statement coerce(2,3.5) returns (2.0,3.5). |
divmod(x, y) | Divides x by y returning a tuple containing the quotient and remainder as derived by long division. This function is effectively equivalent to (a/b, a%b). |
pow(x, y [,z]) | Raises x to the power of y. Note that the return value type is the same as the type of x. This means that you can’t raise an integer to the negative power or raise any number to a power beyond the range of the object type. For example, the statements pow(2,-1) and paw(2,250) both raise exceptions. Similarly, the expression pow(256,(1/2)) returns 1 because the 1/2 calculation rounds down to 0. If the argument z is added, the return value is pow(x,y)%z. but it’s calculated more efficiently. |
round(x [,y]) | Round the floating point numbers to 0 digits, or to y digits after the decimal point if specified. Note that the number returned is still a floating-point number. Use int to convert a floating-point number to an integer, but note that no rounding takes place. |
Conclusion:-
This post has become quite big, I will tell in the next post that string, list, tuple, working with sequence, dictionary, files, object storage, etc. in python. How do they work and how do you use them?
Note:- How did you like this post, do tell by commenting below.
[…] Components of a python program […]