strings tuples and Dictionaries of python
Strings
Strings in Python work differently from those in other scripting languages. Python strings operate in the same basic fashion as C character arrays – a string is a sequence of single characters.
The term sequence is important here because Python gives special capabilities to objects that are based on sequences. Other sequence objects include lists, which are sequences of objects, and tuples, which are immutable sequences of objects. Strings are also immutable; that is, they cannot be changed in place. You’ll see what that really means shortly. Python strings are also your first introduction to the complex objects that Python supports, and they form the basis of many of the other object types that Python supports.
String constants are defined using single or double quotes.
String = Hello World!
String = "Hi there! You're looking great today!"
Note the two formats here there is no difference within Python between using single or double quotes in string constants. By allowing both formats, you can include single quotes in double-quoted constants and double quotes in single-quoted constants without the need to escape individual characters. Python also supports triple-quoted blocks:
helptext="""This is the helptext for an application
that I haven't written yet. It's highly likely that the
help text will incorporate some form of instruction as
to how to use the application which I haven't yet written.
Still, it's good to be prepared!"""
Python continues to add the text to the string until it sees three triple quotes. You can use three single or three double quotes just make sure you use the same number of quotes to start and terminate the constant! Also, you don’t have to follow the normal niles regarding multiline statements, Python incorporates the end-of-line characters in the text unless you use the backslash to join the lines. The preceding, example, when printed, is split onto the same five original lines. To create a single, unbroken paragraph of text, you need to append a backslash to the end of each line, as follows:
helptext = """This is the helptext for an application\
that I haven't written yet. It's highly likely that the\
help text will incorporate some form of instruction as\
to how to use the application which I haven't yet\
written. Still, it's good to be prepared!"""
Putting more than one quoted constant on a line results in the constants being concatenated, such that
String ='I' "am" 'the' "walrus"
Print String
creates the output “Iamthewalrus”. Note the lack of spaces.
You can also concatenate string objects and/or constants using the + operator, just as you would with numbers:
Greeting = 'Hello'
Name = 'Martin'
Print greeting + name
Note , however, that you cannot concatenate strings with other objects. The expression
Print ' Johnny ' + 5
raises an exception because the number object is not automatically translated to a string. There are ways around this _you’ll see some examples shortly. Strings can also be multiplied (repeated) using the operator. For example, the expression
'Coo coo ca choo' = 5
Produces the string
'Coo Coo ca choocoo coo ca choocoo coo ca choocoo coo ca choocoo coo ca choo'
Finally, Python provides the built-in len function which returns the number of characters in a string. The expression
Len(' I am the walrus')
returns the value 15.
The len function actually calculates the length of any object that has a size (lists, dictionaries, etc.).
Strings are just arrays
Strings are, as you’ve already seen, sequences. This means that you can access the individual characters in a string using array notation, and you can access strings as if they were lists.
The array notation for a string follows the same basic format as for other languages; you append square brackets to the variable name, such that:
String = (' I returned a bag of groceries'
Print String[0]
displays the first character of the string Like C, the offset starts at 0 as the first character or element of the string. But unlike C, the indexing also allows you to specify a range, called slicing, and Python is able to determine the position in the string from the end, rather than from the beginning of the string. For example, the script
string = 'I returned a bag of groceries'
print string[0]
print string[2:10]
print string[-1]
print string[-13:]
print string[-9:]
print string[:-9]
Creates the following output:
I
Returned
S
Bag of groceries
Groceries
I returned a bag of
The format of a slice is :
String[start:end]
The processes of indexing and slicing use the following rules:
•The returned string contains all of the characters starting from start up until but not including end.
•If start is specified but end is not, the continues until the end of the string.
•If end is specified but is not, the slice starts from 0 up to but not including end.
•If either start or end, are specified as a negative number, the index specification is taken from the end, rather than from the start of the string where -1 is the last character.
These rules make more sense when used in combination with the diagram shown in Figure 3-1.
You should now be able to determine that the statement
Print String [0]
Prints the first character,
Print String [2:10]
Prints characters 3 through 9,
Print string [-1]
Prints the last character,
Print String [13:]
Prints all of the characters after the fourteenth character,
Print String [-9:]
Print the last nine characters, and
Print String [:-9]
Prints everything except for the last nine characters.
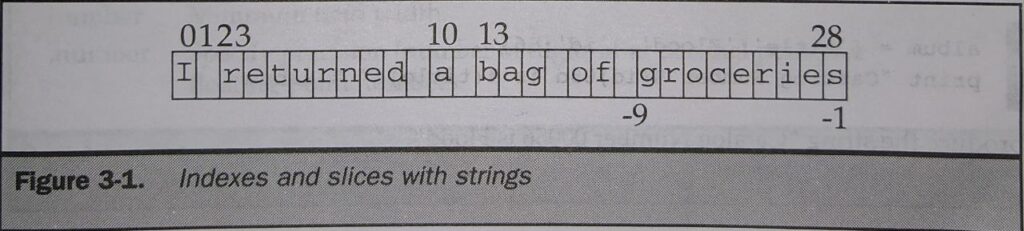
Although python allows you to perform slices and other operations on strings you must remember that strings are immutable sequences- they cannot be changed in place. Therefore, the statement
string[:-9] = 'toffees'
raises an exception. The only ay to change the contents of a string is to actually make a new one. This means that for apparently simple operations such as the one just attempted, you’d have to use the following sequence:
newstring = string[:-9] + 'toffess'
string = newstring
There are, of course, easier ways to change the contents of a string. One way is to use a function supplied in an external module(string) to do the work for us. the other way is to use string formatting, which is discussed in next section.
Tuples
Tuples are, to all intents and purposes, identical to lists except for one detail: tuples are immutable. Unlike lists, which you can chop and change and modify as you like, a tuple is a nonmodifiable list. Once you have created a new tuple, it cannot be modified without making a new tuple.
To create a tuple instead of a list, use parentheses instead of square brackets when defining the tuple contents, as in the following example:
months = ('jan', 'feb', 'mar', 'Apr', 'May', 'jun',
'jul', 'Aug', 'sep', 'oct', 'Nov', 'Dec',)
The preceding statement is actually a very good example of why Python has tuples. Because they cannot be modified, you can use them to store information that you do not want to be altered. In the case of the preceding statement, you are interested in the order of the elements. If you had created this object as a list instead of as a tuple and then accidentally sorted it, the list of months would be largely useless. Without the ordered sequence, the information has no value.
Note that if you try to modify a tuple, an exception is raised to notify you of the error. This can be useful during development and debugging because it helps spot instances where you are misusing an object type.
The following are all examples of tuple use:
One= (1,)
Four = (1,2,3,4)
Five= 1,2,3,4,5
Nest =('Hex', (0,1,2,3,4,5,6,7,8,9), ('A', 'B', 'C', 'D', 'E', 'F'))
The first and third lines in the preceding statements are important to understand. In the case of the first line, to create a one- element tuple you must use the redundant-
looking comma; otherwise, the Python interpreter parses (1) as a constant expression the inclusion of the comma forces Python to interpret the statement as a tuple.
The third line shows tuple creation without the use of parentheses Python allows you to create a tuple without using parentheses in those situations where the lack of parentheses is not ambiguous. In this instance, it’s obvious you are creating a tuple. As a general rule, you should use parentheses to highlight to you and any other programmers looking at your code that you are introducing a tuple.
Using Tuples
Tuples support the same basic operations as lists. You can index, slice, concatenate, and repeat tuples just as you can lists. The len function also works since a tuple is a sequence. The only actions you can’t perform with a tuple are to modify them or to perform any of the methods available to lists since these methods make modifications. It’s also worth noting again that tuple operations return tuples. The statement
three= five[:3]
makes three a tuple, not a list.
Some functions also return tuples rather than lists, and some functions even require tuples as arguments. See “Type Conversions for information on translating between Python object types.
Working with Sequences
The object types of strings, lists, and tuples belong to a generic Python object class called sequences. A sequence object is any object whose elements can be accessed using a numbered index. In the case of a string, each character is available via the index; for lists, it’s each object. Because sequences have an order and are made up of one or more elements, there are occasions when you want to use the list as either a sequence or a traditional list of values to use as a reference point. Python provides a single operator, in that allows you to access the contents of a list in a more logical fashion.
Membership
If you are working with a list of possible values for a particular structure and want to verify the existence of a single element within the list, there are normally two choices available to you. Either you write a function to work through the contents of the list looking for the object, or you use a superior data structure such as a dictionary to store the item and then use the object’s built-in methods to determine whether the object is a member of your pseudo-list.
Within python, you can use the in operator to determine whether a single object is a member of the list, returning 1 if the element is found, as in the following example:
>>> List = [1, 2, 3]
>>> 1 in list
1
>>> 4 in list
0
This process also works with Strings :
>>> Word = 'supercalifragilisticexpialidociuos'
>>> 'x' in word
1
And Tuples:
>>> Days= ('mon', 'tue', 'wed', 'thu' , 'fri', 'sat', 'sun' )
>>> 'mon' in days
1
Iteration
You haven’t yet learned about the loop operators supported by Python. Most of the usual operations of while and for are supported, and we can also iterate through the individual elements of a sequence. Again, Python uses the in operator, which this time returns each element of the sequence to each iteration of the loop:
For day in arrays:
Print day
You’ll see more examples of this later in this chapter and throughout the rest of the book.
Dictionaries
A dictionary is an associative array; instead of using numbers to refer to individual elements of the list, you use other objects-generally strings. A dictionary element is split into two parts, the key and the value. You access the values from the dictionary by specifying the key.
Like other objects and constants, Python uses a strict format when creating dictionaries:
Monthdays = {'jan' : 31, 'feb' : 28, 'mar' : 31,
'apr' : 30, 'may':31, 'jun' : 30,
'jul' : 31, 'aug' :31, 'sep' : 30,
'oct' : 31, 'Nov': 30, 'Dec' : 31}
You can also nest dictionaries, as in the following example:
album= {'Flood' : {1:' Birdhouse in your soul'}}
To access an entry you use the square bracket to define the index entry of the element yu want t be returned, just as you do with strings or lists:
print 'january has' ,monthdays ['jan'], 'days'
print 'first track is' , albumas ['flood'] [1]
However, the interpreter raises an exception if the specified key cannot be found. see the section “Dictionary Methods” later in this chapter for details on the get and has_key methods. Again, just like lists, you can modify an existing key/value pair by redefining the entry in place:
monthdays['Feb'] = 29
If you had specified a different key, you would have added rather than updated the entry. The following example
monthdays['feb'] =29
introduce a thirteenth element to the dictionary.
you can delete entries entries in a dictionary using the del function:
del monthdays['feb']
Dictionaries are not sequences, so concatenation and multiplication will not work–
if you try them, both operations will raise an exception.
Using Dictionaries
Although dictionaries appear to work just like lists, the two are in fact very different. Dictionaries have no order-like lists, the indexes (keys) must be unique but there is no logical order to the keys in the dictionary. This means that a dictionary is not a sequence; you cannot access the entries in a dictionary sequentially in the same way you can access entries in a string, list, or tuple. This also has the unpleasant side effect
that when accessing the same dictionary at two separate times, the order in which the information is returned by the dictionary does not remain constant.
However, the dictionary object provides two methods, keys and values, that return a list of all the keys and values defined within the dictionary. For example, to get a list of months from the dictionary of months in the earlier example, use the following statement:
months = monthdays.keys()
The months object is now a list of the month strings that formed the keys of the monthsdays dictionary.
len (monthdays)
returns the correct value of 12.
Dictionary Methods
Table 3-10 lists the full list of the methods supported by the dictionary object type.
Method | Description |
has_key(x) | Return true if the dictionary has the key x. |
keys() | Returns a lost of keys. |
values() | Returns a list of values. |
dict.items() | Return a list of tuples; each tuple consists of a key and its corresponding value from the dictionary. |
clear() | Removes all the items from the dictionary. |
copy() | Return a copy f the top level of the dictionary, but does not copy nested structures; only copies the references to those structures, See the section “Nesting Objects.” |
update(x) | Updates the contents of the dictionary with the key/value pairs from the dictionary x. Note that the dictionaries are merged, not concatenated, since you can’t have duplicate keys in a dictionary. |
get(x[,y]) | Returns the key x or None if the key cannot be found, and can therefore be used in place of dict[x]. if y is supplied, this method returns that value if x is not found. |
Sorting Dictionaries
The keys() and values() methods to a dictionary object return a list of all the keys or values within the dictionary. This useful when iterating over a dictionary or when using dictionary for de – duplicating values. However, we cannot nest the keys() or values() and the corresponding list of tuple sort() methods into one call. To get a sorted not, it’s a two-stage process:
Months = monthdays.keys()
Months.sort()
Many users try the following:
Months = monthdays. Keys ().sort()
But that won’t work. The reason is quite simple the sort method modifies a list in places it doesn’t return the new list. Although the object generated by the keys method is sorted, the information is never returned and when the statement ends, the temporary sorted list i object is destroyed, while months contains the special value None.
The problem with this method is not really apparent until you try to let the contents of a dictionary in an ordered fashion. You have to use the two-stage method shown in the first example of sorting a dictionary:
Keys = monthdays.keys ()
Keys.sort()
For key in keys:
...
If you want to sort the list based on the values rather than on the keys, the process get even more complicated. You can’t access the information in a dictionary using values only using the keys. What you need to do is sort a list of tuple pairs by supplying a custom compaction function to the sort method of a list.
You can get a list of tuple pairs using the items method. The process looks something like this:
Monthdays = {'jan' : 31, 'feb' : 28, 'mar' : 31,
'apr' : 30, 'may':31, 'jun' : 30,
'jul' : 31, 'aug' :31, 'sep' : 30,
'oct' : 31, 'Nov': 30, 'Dec' : 31}
Months=monthdays.items()
Months.sort(lambda f, s: cmp(f[1], s[1]))
For month, days in months:
Print 'There are' , days, 'days in' , month
We haven’t covered many of the techniques used in the above example, but here’s how it works. Line 5 in the preceding example gets a list of the key/value pairs as tuples Line 6 sorts the list by comparing the value component of each tuple; the lambda is an anonymous function. (See Chapter 4 for more information on the lambda function and see Chapter 7 for an explanation of the custom element to the sort method.) Then the far loop extracts the month and number of days in each individual tuple from the list of tuples before printing each one.
When executed, the script output looks like this:
There are 28 days in Feb
There are 30 days in Nov
There are 30 days in Apr
There are 30 days in Jun
There are 30 days in Sep
There are 31 days in May
There are 31 days in Aug
There are 31 days in Oct
There are 31 days in Jul
There are 31 days in Jan
There are 31 days in Dec
There are 31 days in Mar
Dictionaries are one of the most useful storage elements because they enable you to access information using anything as a key-Python allows you to use any object as a key to the key/value pair. Chapter 10 takes a closer look at dictionaries in Python.
Conclusion:-
This post has become quite big, I will tell in the next post that file, object storage, type conversion, type comparisons, statement, statement format, control statements, etc. in python. How do they work and how do you use them?
Note:- How did you like this post, do tell by commenting below.
[…] post has become quite big, I will tell in the next post that string, list, tuple, working with sequence, dictionary, files, object storage, etc. in python. How do they […]
[…] Strings, List, Tuples, Dectionaries […]