How to check a key exists in JavaScript
The ability to determine the presence or absence of specific keys within objects and components within arrays is critical for successful data manipulation and decision-making in JavaScript programming. Whether you’re working with complicated data structures or conducting simple data validation, the ability to verify for the existence of keys and elements gives you the tools you need to write robust and dependable code.
We will look at how to use built-in functions like hasOwnProperty(), the in operator, indexOf(), and the newer includes() method to accomplish these tests. These techniques are critical in improving the efficiency and reliability of your code, allowing you to interact with data with confidence while avoiding errors and unexpected behaviour.
Various techniques in JavaScript can be used to determine whether a key exists in an object or a specific property exists in an array. Here’s how to go about it:
Checking Object Keys:
To see if an object has a key, use the hasOwnProperty() function or the ‘in’ operator.
Method 1 :- Using ‘in’ operator
In JavaScript, the ‘in’ operator is used to determine whether a specific property (key) exists in an object. It is typically used to determine whether a specific key exists as a property in an object, regardless of its value.
Syntax :- propertyName in object |
Here Are the Code:-
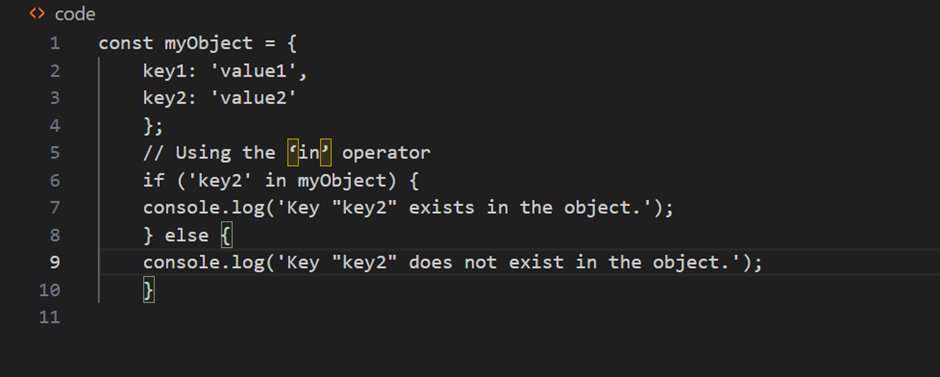
const myObject = {
key1: 'value1',
key2: 'value2'
};
// Using the in operator
if ('key2' in myObject) {
console.log('Key "key2" exists in the object.');
} else {
console.log('Key "key2" does not exist in the object.');
}
Example:– This example uses “in” operator to check the existence of key in JavaScript object.
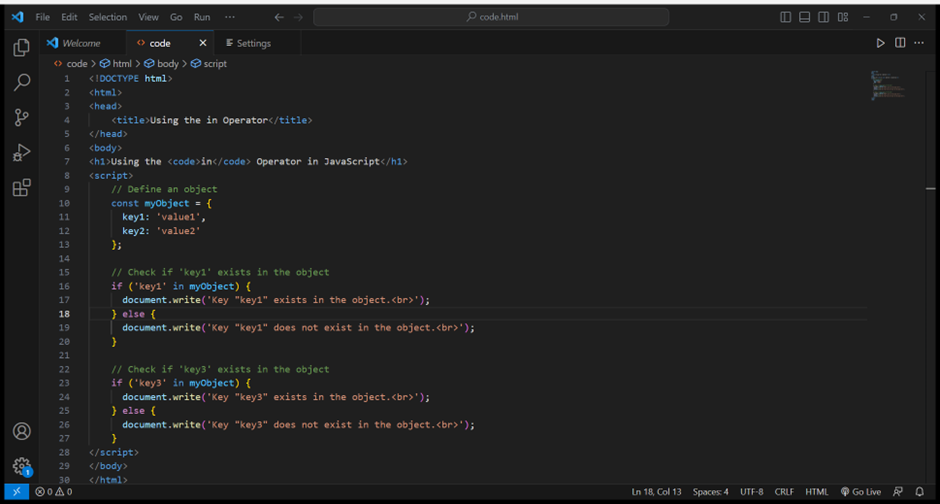
<!DOCTYPE html>
<html>
<head>
<title>Using the in Operator</title>
</head>
<body>
<h1>Using the <code>in</code> Operator in JavaScript</h1>
<script>
// Define an object
const myObject = {
key1: 'value1',
key2: 'value2'
};
// Check if 'key1' exists in the object
if ('key1' in myObject) {
document.write('Key "key1" exists in the object.<br>');
} else {
document.write('Key "key1" does not exist in the object.<br>');
}
// Check if 'key3' exists in the object
if ('key3' in myObject) {
document.write('Key "key3" exists in the object.<br>');
} else {
document.write('Key "key3" does not exist in the object.<br>');
}
</script>
</body>
</html>
The HTML file in this sample contains JavaScript code contained within script> tags. The JavaScript code creates an object called myObject, which has the values ‘key1’ and ‘key2’. It then checks the object for the presence of ‘key1’ and ‘key3’ using the in operator. The document.write() method is used to display the results of these checks on the webpage.
When you open this HTML file in a web browser, the result will show if the keys are present in the object or not.
Method 2:- Using the hasOwnProperty() method
The hasOwnProperty() method is a JavaScript built-in method that determines whether an object has a given property (key). This function determines whether the supplied property is a direct property of the object, which means it was not inherited from the prototype chain.
Syntax – object.hasOwnProperty(propertyName) |
Here are the codes:-
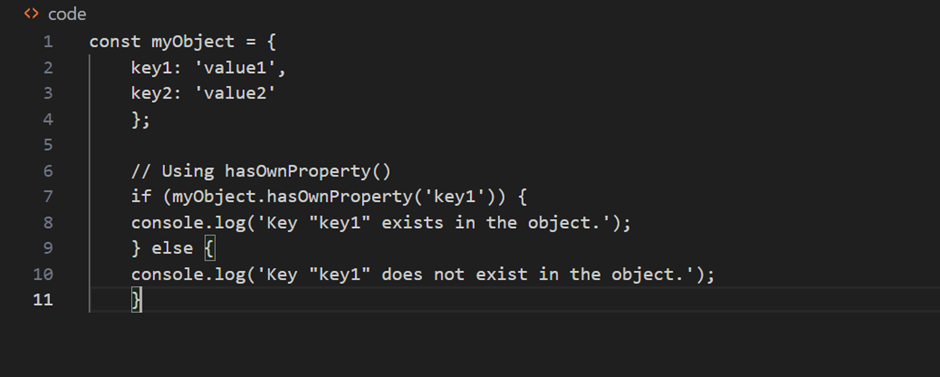
const myObject = {
key1: 'value1',
key2: 'value2'
};
// Using hasOwnProperty()
if (myObject.hasOwnProperty('key1')) {
console.log('Key "key1" exists in the object.');
} else {
console.log('Key "key1" does not exist in the object.');
}
Example: Here are the example that uses “object.hasOwnProperty” operator to check the existence of key in JavaScript object.
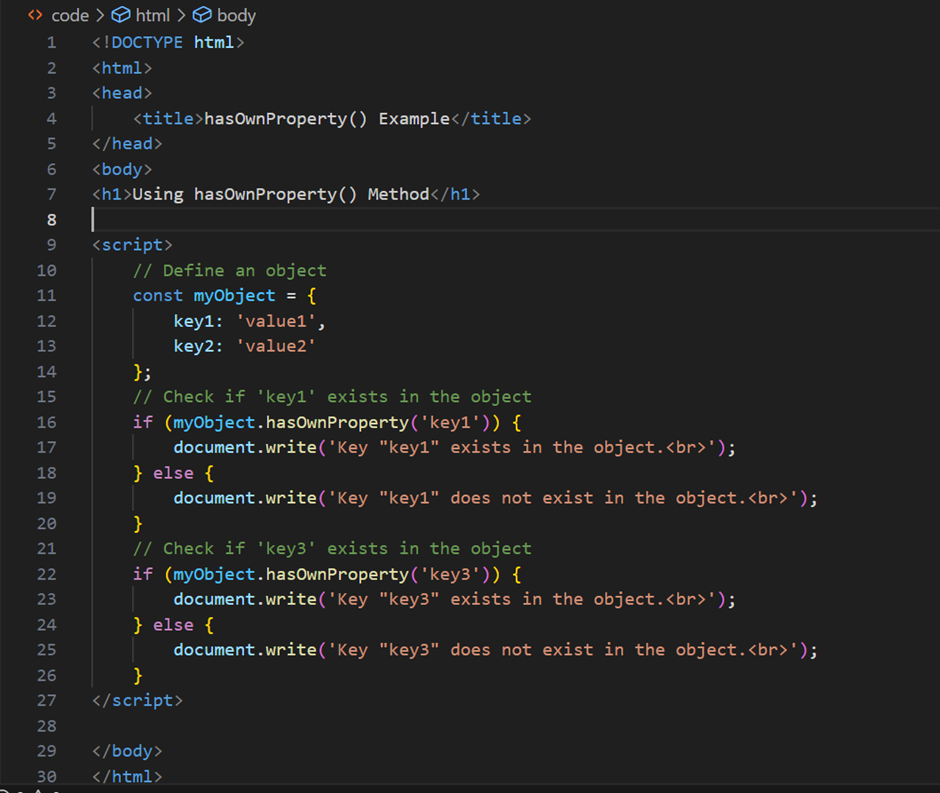
<!DOCTYPE html>
<html>
<head>
<title>hasOwnProperty() Example</title>
</head>
<body>
<h1>Using hasOwnProperty() Method</h1>
<script>
// Define an object
const myObject = {
key1: 'value1',
key2: 'value2'
};
// Check if 'key1' exists in the object
if (myObject.hasOwnProperty('key1')) {
document.write('Key "key1" exists in the object.<br>');
} else {
document.write('Key "key1" does not exist in the object.<br>');
}
// Check if 'key3' exists in the object
if (myObject.hasOwnProperty('key3')) {
document.write('Key "key3" exists in the object.<br>');
} else {
document.write('Key "key3" does not exist in the object.<br>');
}
</script>
</body>
</html>
In this examples the code checks for the existence of key 1 and key 3 in the ‘myobject’ object. When you run this HTML file in web browser, you will see the output that signifies the usage of the ‘hasOwnProperty() method for checking object property.
Checking Array Element
In JavaScript, checking array elements refers to the act of determining whether a certain value exists within an array. Arrays are ordered lists of values, and detecting if a specific value exists in an array is a typical programming task.
There are various methods to check if elements exist in the array. Some of them are discussed here:-
Using the “indexof()” method :-
The indexOf() method returns the index of the array’s first occurrence of a supplied value. If the value cannot be discovered, the function returns -1.
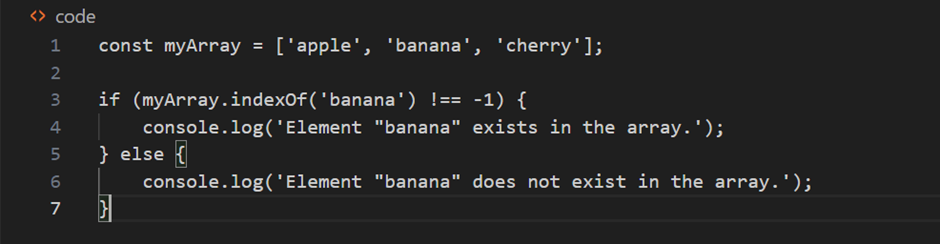
const myArray = ['apple', 'banana', 'cherry'];
if (myArray.indexOf('banana') !== -1) {
console.log('Element "banana" exists in the array.');
} else {
console.log('Element "banana" does not exist in the array.');
}
Using the “include()” method:-
The includes() method checks whether an array contains a particular element. If the element is found, it returns true; otherwise, it returns false.
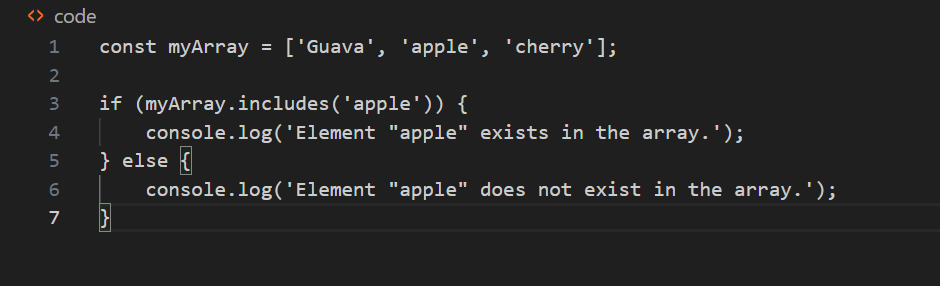
const myArray = ['Guava', 'Apple', 'cherry'];
if (myArray.includes('Apple')) {
console.log('Element "Apple" exists in the array.');
} else {
console.log('Element "Apple" does not exist in the array.');
}
Using the “find()” method:-
The find() method returns the first entry in an array that meets a testing function.
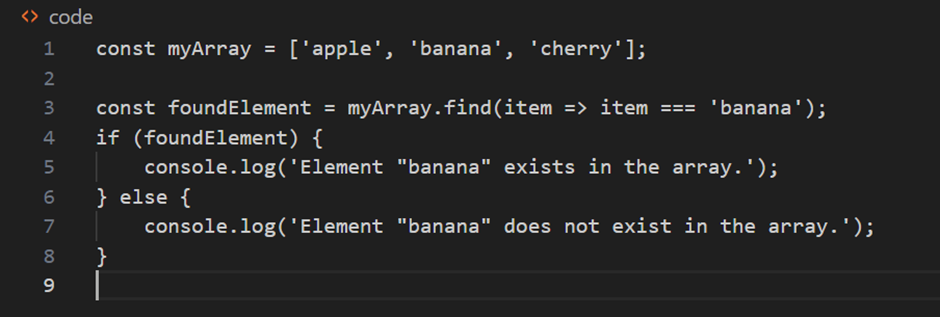
const myArray = ['apple', 'banana', 'cherry'];
const foundElement = myArray.find(item => item === 'banana');
if (foundElement) {
console.log('Element "banana" exists in the array.');
} else {
console.log('Element "banana" does not exist in the array.');
}
Using the “some()” method:-
The some() method determines whether at least one array element passes the test implemented by the provided function.
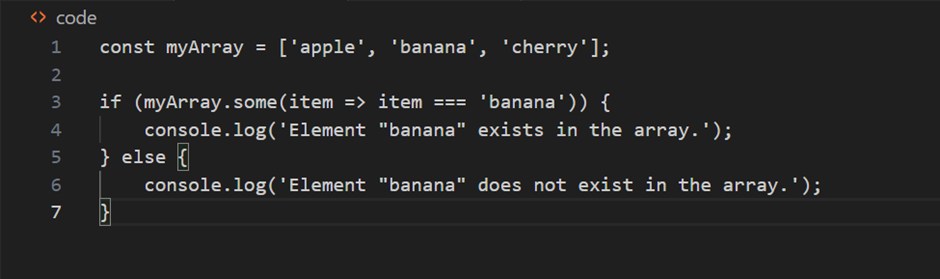
const myArray = ['apple', 'banana', 'cherry'];
if (myArray.some(item => item === 'banana')) {
console.log('Element "banana" exists in the array.');
} else {
console.log('Element "banana" does not exist in the array.');
}
So far we have discussed the various methods to find the key exist in an object in javascript. The first way is compare the key with the undefined so if it is undefined then it is the key is not exist. From the above discussion we have gone through various methods that makes task easier to perform it having some piece of code only.
Using the “in” Operator we determines whether a given property (key) exists in an object or not. It is especially useful for checking keys in objects and browsing their properties.
Using the ” hasOwnProperty()” Method, we are able to determines whether a property is a direct property of an object or not by without taking out the properties that are inherited from prototypes. It comes in especially when working with objects and their immediate characteristics.
When working with arrays, we discussed some of the functions such as indexOf(), includes(), find(), and some() that provides most significant ways to check for the existence of elements. These methods helps in the manipulation and sometimes controlling the behaviour of our program.
Conclusion
Verifying the existence of keys in JavaScript programming is a highly critical task. It assists in executing code smoothly and without any errors. It also ensures that users are validating the presence of specific elements, which hold significance, to check the existence of data or any information. This validation holds even greater importance.