Python control statements with examples
Control StatementsÂ
Python processes program statements in sequence until the program states otherwise through the use of a control statement or a function call. Python supports three different control statements, if, for, and while. The if statement is the most basic control statement: it selects a statement block to execute based on the result of one or more expressions. Both for and while are loop statements.
If
The if statement accepts an expression and then executes the specified statements if the statement returns true. The general format for the if statement is:
If EXPRESSION:
BLOCK
elif EXPRESSION2:
BLOCK2
else:
BLOCK3
The EXPRESSION is the test you want to perform (see Table 3-12), a result of true means that the statements in BLOCK are being executed. The optional elif statement performs further tests when EXPRESSION fails, executing BLOCK2 if EXPRESSION2 returns true or executing BLOCK3 otherwise. You can have multiple elif statements within a single if statement. If no expressions match, then the optional block to the else statement is executed.
Note that in each case, the preceding line to the statement block ends with a colon. This indicates to Python that a new statement block should be expected. Unlike many other languages, Python is a little more relaxed about its definitions of the code block. Both C and Perl use curly brackets (or braces) to define the start and end of a block. Python uses indentation-any lines after the colon must be indented (to the same level) if you want them to be in the same logical code block.
You can see how the indentation and blocks work by looking at Figure 3-2. Notice in this figure that Block 1 continues after the indentations for Block 2 and Block 3 no longer exist. See the rest of the script for details on the block definitions and indentation.
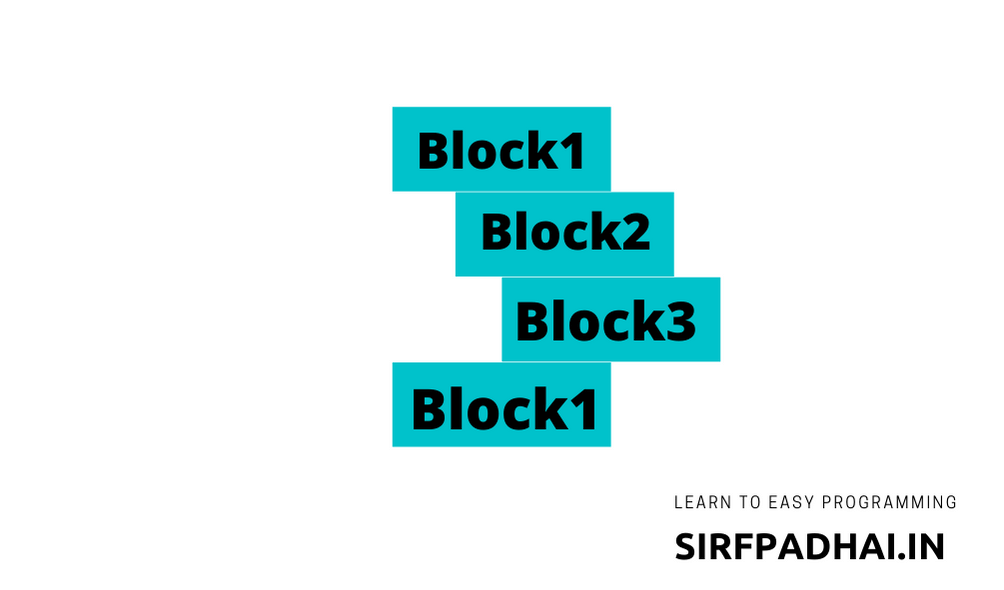
Consider the following example combining if and elif statement:
If (result = = 1):
Print 'Got a one'
elsif (result>1):
Print 'Got more than one'
else:
Print 'Got something else'
For simple test, you can combine the statement and block onto a single line:
If (1): print 'Hello World'
This really only work for single-line block.
Note:- If you want to perform a switch or case statement in python, you must use an if statement with multiple elif expressions.
While
The while loop accepts a single expression and the loop continues to iterate as long as the test continues to return true. The expression is reevaluated before each iteration.
The basic format is therefore:
While EXPRESSION :
BLOCK
else:
BLOCK
For example, to work through the characters in a string, you might use
String = 'I palindrome I'
While(len (string)):
Char = string [0]
String = String [1:]
Print 'Give me a' , char
Which outputs:-
Give me a I
Give me a
.....
Give me a
Give me a I
Note that in this particular example, it’s probably easier to use a for loop. The optional else block is only executed I’d the loop exits when EXPRESSION returns false, rather than the execution being broken by the break statement. See the example of a for loop in the next subsection.
ForÂ
The for loop is identical to the list form of the for loop in perl. The basic format for the for loop is:
For TARGET in OBJECT:
BLOCK
Else :
BLOCK
You specify the object to be used as the iterator within the loop and then supply any form of sequence object to iterate through.
For example:-
For number in [1,2,3,4,5,6,7,8,9] :
Print 'I can count to ', number
For each iteration of the loop, number is set to the next value within the array producing the output
I can count to 1
I can count to 2
.....
I can count to 8
I can count to 9
Because it works with any Sequence, you can also work through strings as in
For letter in 'Martin' :
Print 'Give me a', letter
Which generates
Give me a M
Give me a a
Give me a r
Give me a t
Give me a i
Give me a n
In addition, just like the while loop, the else statement block is executed when the loop exits normally as in
For number in [1,3,5,7]:
If number > 8 :
Print "I can't work with numbers that are higher than 8!"
Break
Print '%d squared is %d' %(number, pow(number ,2))
else:
Print 'Made it!'
Which outputs:-
1 squared is 1
3 squared is 9
5 squared is 25
7 squared is 49
Made it!
Ranges
Because the for loop does not support the loop counter format offered by Perl and C, you need to use a different method to iterate through a numerical range. There are two possible methods. Either use the while loop:
While (x <10):
Print x
x =x+1
Alternatively, you can use the range function to generate a list of values:
For x in range (10):
....
The range function returns a list. The basic format of the range function is
Range([start, ] stop [,step])
In its single-argument form, range returns a list consisting of each number up to, but not including, the number supplied:
>>> range (10)
[ 0,1,2,3,4,5,6,7,8,9]
If the range function is supplied two arguments, it returns a list of numbers starting at the first argument, up to but not including the last argument:
>>>range (4,9)
[4,5,6,7,8]
The final form, with three arguments, allows you to define the intervening step:
>>> range(0,10,2)
[0,2,4,6,8]
Because the range function returns a list, it can create, for very large ranges, very large lists that require vast amounts of memory to store. To get around this, use the xrange function. The xrange function has the exactly format and indeed, performs exactly the same function as range, but it does not create an intervening list and therefore requires less memory for very large ranges. On the other hand, it produces a very large list that results in a processing overhead as new objects are created and added to the list structure.
Loop Control Statements
Python supports three loop control statements that modify the normal execution of a loop. They are break continue and pass. The break statement exits the current loop, ignoring any else statement, continuing execution after the last line in the loop’s statement block.
The continue statement immediately forces the loop to proceed to the next iteration, ignoring any remaining statements in the current block. The loop statement expression is reevaluated. The example
a = 20
while (x):
x = x-1
If not x % 3: continue
Print x, 'is not a multiple of 3'
generates the following output:
19 is not a multiple of 3
17 is not a multiple of 1
16 is not a multiple of 3
4 is not a multiple of 3
2in not a multiple of 3
1 is not a multiple of 3
The pass statement is effectively a no-op-it does nothing. The statement
if (1): pass
does nothing at all.
Although it may seem pointless, there are times when you want to be able to identify a specific event, but ignore it. This is especially true of exceptions, the Python error-handling system. You’ll see pass and exceptions in action in Chapter 5.
Common Traps
For the programmer migrating to Python, there are a number of common trap that trick the unwary. Most relate to the very minor differences between Python and other languages, particularly if you have been used to Perl or Shellscript.
Variable Names
Variables within Python follow these basic rules and differences from other languages:
•You do not need to predeclare variables before they are used; Python sets the object type based on the value it is assigned.
•Variables are given simple alphanumeric names.
•Variables do not require qualification using special characters.
•Some variables have embedded methods that operate on the object’s contents.
Blocks and Indentation
Blocks start after a colon on the preceding line. For example:
if (1):
print 'I am a new block'
The block continues until a new block is created, or until the indentation for the block ends.
When indenting, use either tabs or spaces__ do not mix the two because this is likely to confuse the Python interpreter and people maintaining the code.
Method Calls
The most common error with a method call to an object is to assume that the call returns information. The statement
sorted = list.sort()
sorts the object list, but sets the value of sorted to the special None.
[…] control statements with examples(if, while, for, Loop control) […]